How to Make an Android PDF Viewer in Java
This section will help you to quickly get started with ComPDFKit PDF SDK to make an Android app in Java with step-by-step instructions. Through the following steps, you will get a simple application that can display the contents of a specified PDF file.
Create a New Project
- Use Android Studio to create a Phone & Tablet project. Here we create a No Activity project.
Add the ComPDFKit PDF SDK Package
The first step we need to take is to import the ComPDFKit PDF SDK. You can integrate it in two ways: using Integrate With Gradle
or Integrate Manually
.
Integrate With Gradle
- Open the
settings.gradle
file located in your project's root directory and add themavenCentral
repository:
dependencyResolutionManagement {
repositoriesMode.set(RepositoriesMode.FAIL_ON_PROJECT_REPOS)
repositories {
google()
+ mavenCentral()
}
}
- Open the
build.gradle
file in the application module directory:
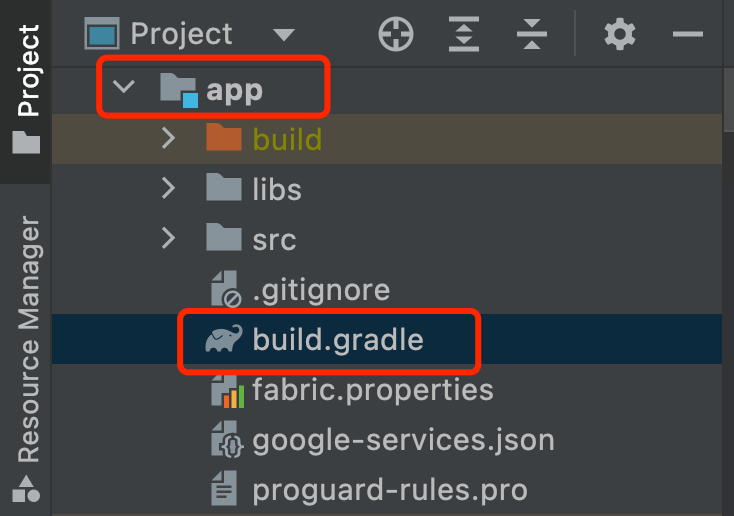
Edit it and add the complete ComPDFKit SDK
dependency:
dependencies {
implementation 'com.compdf:compdfkit:2.4.0'
implementation 'com.compdf:compdfkit-ui:2.4.0'
}
- Apply for read and write permissions in
AndroidManifest.xml
:
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/>
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE"/>
Note: On your apps that target Android 6.0 or higher, make sure to check for and request read and write permissions to external storage at runtime.
- If you use an online license, please add network access permissions in
AndroidManifest.xml
:
<uses-permission android:name="android.permission.INTERNET"/>
Integrate Manually
- Copy "ComPDFKit.aar" and "ComPDFKit-UI.aar" to the "libs" directory of the app.
- Add the following code into the app dictionary’s "build.gradle" file:
...
dependencies {
/*ComPDFKit SDK*/
implementation(fileTree('libs'))
...
}
...
- Add ComPDFKit PDF SDK for Android as a dependency to the project. Inside the app dictionary’s "build.gradle", add "ComPDFKit.aar", "ComPDFKit-UI.aar", and the related support libraries to the
dependencies
. For simplicity, update the dependencies as follows:
dependencies {
...
//glide
implementation 'com.github.bumptech.glide:glide:4.12.0'
annotationProcessor 'com.github.bumptech.glide:compiler:4.12.0'
implementation 'androidx.documentfile:documentfile:1.0.1'
}
- Apply for read and write permissions in
AndroidManifest.xml
:
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/>
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE"/>
Note: On your apps that target Android 6.0 or higher, make sure to check for and request read and write permissions to external storage at runtime.
- If you use an online license, please add network access permissions in
AndroidManifest.xml
:
<uses-permission android:name="android.permission.INTERNET"/>
Apply the License Key
Add this license in the AndroidManifest.xml of the main module. In version 1.13.0, we introduced a brand-new online authentication license scheme for ComPDFKit SDK. By default, the SDK performs online authentication. If you are using a version prior to 1.13.0, please refer to the following example to configure the SDK for offline authentication mode:
- Online license
<!-- Each ComPDFKit license is bound to a specific applicationId -->
<!-- For example: com.compdfkit.pdfviewer -->
<meta-data
android:name="compdfkit_key_online"
android:value="Your ComPDFKit Key" />
You can also initialize ComPDFKit SDK in code using:
CPDFSdk.init(context, "your compdfkit license", false);
- Offline license
<!-- Each ComPDFKit license is bound to a specific applicationId -->
<!-- For example: com.compdfkit.pdfviewer -->
<meta-data
android:name="compdfkit_key"
android:value="Your ComPDFKit Key" />
You can also initialize ComPDFKit SDK in code using:
CPDFSdk.init(context, "your compdfkit license");
Add Proguard Rules
In the proguard-rules.pro
file, please add the obfuscation configuration information for compdfkit
as follows:
-keep class com.compdfkit.ui.** {*;}
-keep class com.compdfkit.core.** {*;}
Display a PDF Document
- Copy a PDF document into the assets directory of your Android project. For example, import the file "Quick Start Guide.pdf" to the path src/main/assets.
- Create a new Empty Activity under your package, and set the activity name to MainActivity.
Android Studio will automatically generate a source file called "MainActivity.java" and a layout file called "activity_main.xml".
The source file:
The layout file:
- Create a
CPDFReaderView
in your "activity_main.xml" to display the contents of the PDF document:
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<!-- Create a CPDFReaderView -->
<com.compdfkit.ui.reader.CPDFReaderView
android:id="@+id/readerview"
android:layout_width="match_parent"
android:layout_height="match_parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
Get the CPDFReaderView
from the layout or create a CPDFReaderView
directly in the code in the corresponding MainActivity.java file:
// Your MainActivity.java file
package com.compdfkit.pdfviewer;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import com.compdfkit.ui.reader.CPDFReaderView;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Get CPDFReaderView from xml.
CPDFReaderView readerView = findViewById(R.id.readerview);
// Code to create CPDFReaderView.
// CPDFDocument readerView = new CPDFReaderView(content);
}
}
// Your MainActivity.kt file
package com.compdfkit.pdfviewer
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import com.compdfkit.ui.reader.CPDFReaderView
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// Get CPDFReaderView from xml.
val readerView = findViewById<CPDFReaderView>(R.id.readerview)
// Code to create CPDFReaderView.
// CPDFDocument readerView = new CPDFReaderView(content);
}
}
- Open the document. This is a time-consuming process, so it needs to be executed in a sub-thread. After the document is opened successfully, the UI that renders the PDF is initiated:
// Your MainActivity.java file
... //imports
public class MainActivity extends AppCompatActivity {
// Copy the PDF file from the assets folder to the cache folder.
private void copyPdfFromAssetsToCache(String fileName) {
try {
InputStream inputStream = getAssets().open(fileName);
File outputFile = new File(getCacheDir(), fileName);
FileOutputStream outputStream = new FileOutputStream(outputFile);
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
}
inputStream.close();
outputStream.flush();
outputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
CPDFReaderView readerView = findViewById(R.id.readerview);
// Code to create CPDFReaderView.
// CPDFDocument readerView = new CPDFReaderView(content);
//Create a document object.
CPDFDocument document = new CPDFDocument(this);
new Thread(() -> {
String fileName = "Quick Start Guide.pdf";
copyPdfFromAssetsToCache(fileName);
File file = new File(getCacheDir(), fileName);
String filePath = file.getAbsolutePath();
//Open document.
CPDFDocument.PDFDocumentError error = document.open(filePath);
if (error == CPDFDocument.PDFDocumentError.PDFDocumentErrorPassword) {
//The document is encrypted and requires a password to open.
error = document.open(filePath, "password");
}
if (error == CPDFDocument.PDFDocumentError.PDFDocumentErrorSuccess) {
//The document is opened successfully and data can be parsed and manipulated.
} else {
//The PDF file failed to open. You can refer to the API file for specific error
}
}).start();
}
}
// Your MainActivity.kt file
... //imports
class MainActivity : AppCompatActivity() {
// Copy the PDF file from the assets folder to the cache folder.
private fun copyPdfFromAssetsToCache(fileName: String) {
try {
val inputStream = assets.open(fileName)
val outputFile = File(cacheDir, fileName)
val outputStream = FileOutputStream(outputFile)
val buffer = ByteArray(1024)
var bytesRead: Int
while (inputStream.read(buffer).also { bytesRead = it } != -1) {
outputStream.write(buffer, 0, bytesRead)
}
inputStream.close()
outputStream.flush()
outputStream.close()
} catch (e: IOException) {
e.printStackTrace()
}
}
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val readerView = findViewById<CPDFReaderView>(R.id.readerview)
// Code to create CPDFReaderView.
// CPDFDocument readerView = new CPDFReaderView(content);
//Create a document object.
val document = CPDFDocument(this)
Thread {
val fileName = "Quick Start Guide.pdf"
copyPdfFromAssetsToCache(fileName)
val file = File(cacheDir, fileName)
val filePath = file.absolutePath
//Open document.
var error = document.open(filePath)
if (error == CPDFDocument.PDFDocumentError.PDFDocumentErrorPassword) {
//The document is encrypted and requires a password to open.
error = document.open(filePath, "password")
}
if (error == CPDFDocument.PDFDocumentError.PDFDocumentErrorSuccess) {
//The document is opened successfully and data can be parsed and manipulated.
} else {
//The PDF file failed to open. You can refer to the API file for specific error
}
}.start()
}
}
- Set the basic properties of
CPDFReaderView
:
// Your MainActivity.java file
... // imports
public class MainActivity extends AppCompatActivity {
// Create a handler to run the code on the main thread.
private Handler mainThreadHandler = new Handler(Looper.getMainLooper());
...
if (error == CPDFDocument.PDFDocumentError.PDFDocumentErrorSuccess) {
// The document is opened successfully and data can be parsed and manipulated.
mainThreadHandler.post(() -> {
// Set the document content for UI.
readerView.setPDFDocument(document);
});
} else {
// The PDF file failed to open. You can refer to the API file for specific error
}
...
}
// Your MainActivity.kt file
... // imports
public class MainActivity : AppCompatActivity() {
// Create a handler to run the code on the main thread.
private var mainThreadHandler = Handler(Looper.getMainLooper());
...
if (error == CPDFDocument.PDFDocumentError.PDFDocumentErrorSuccess) {
// The document is opened successfully and data can be parsed and manipulated.
mainThreadHandler.post {
// Set the document content for UI.
readerView.setPDFDocument(document);
}
} else {
// The PDF file failed to open. You can refer to the API file for specific error
}
...
}
- Your code may resemble the following at this stage:
// Your MainActivity.java file
... // imports
public class MainActivity extends AppCompatActivity {
// Create a handler to run the code on the main thread.
private Handler mainThreadHandler = new Handler(Looper.getMainLooper());
// Copy the PDF file from the assets folder to the cache folder.
private void copyPdfFromAssetsToCache(String fileName) {
try {
InputStream inputStream = getAssets().open(fileName);
File outputFile = new File(getCacheDir(), fileName);
FileOutputStream outputStream = new FileOutputStream(outputFile);
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
}
inputStream.close();
outputStream.flush();
outputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
CPDFReaderView readerView = findViewById(R.id.readerview);
//Create a document object.
CPDFDocument document = new CPDFDocument(this);
new Thread(() -> {
String fileName = "Quick Start Guide.pdf";
copyPdfFromAssetsToCache(fileName);
File file = new File(getCacheDir(), fileName);
String filePath = file.getAbsolutePath();
//Open document.
CPDFDocument.PDFDocumentError error = document.open(filePath);
if (error == CPDFDocument.PDFDocumentError.PDFDocumentErrorPassword) {
//The document is encrypted and requires a password to open.
error = document.open(filePath, "password");
}
if (error == CPDFDocument.PDFDocumentError.PDFDocumentErrorSuccess) {
//The document is opened successfully and data can be parsed and manipulated.
mainThreadHandler.post(() -> {
//Set the document to the reader view.
readerView.setPDFDocument(document);
});
} else {
//The PDF file failed to open. You can refer to the API file for specific error
}
}).start();
}
}
// Your MainActivity.kt file
... // imports
class MainActivity : AppCompatActivity() {
// Create a handler to run the code on the main thread.
private val mainThreadHandler = Handler(Looper.getMainLooper())
// Copy the PDF file from the assets folder to the cache folder.
private fun copyPdfFromAssetsToCache(fileName: String) {
try {
val inputStream = assets.open(fileName)
val outputFile = File(cacheDir, fileName)
val outputStream = FileOutputStream(outputFile)
val buffer = ByteArray(1024)
var bytesRead: Int
while (inputStream.read(buffer).also { bytesRead = it } != -1) {
outputStream.write(buffer, 0, bytesRead)
}
inputStream.close()
outputStream.flush()
outputStream.close()
} catch (e: IOException) {
e.printStackTrace()
}
}
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val readerView = findViewById<CPDFReaderView>(R.id.readerview)
//Create a document object.
val document = CPDFDocument(this)
Thread {
val fileName = "Quick Start Guide.pdf"
copyPdfFromAssetsToCache(fileName)
val file = File(cacheDir, fileName)
val filePath = file.absolutePath
//Open document.
var error = document.open(filePath)
if (error == PDFDocumentError.PDFDocumentErrorPassword) {
//The document is encrypted and requires a password to open.
error = document.open(filePath, "password")
}
if (error == PDFDocumentError.PDFDocumentErrorSuccess) {
//The document is opened successfully and data can be parsed and manipulated.
mainThreadHandler.post {
//Set the document to the reader view.
readerView.pdfDocument = document
}
} else {
//The PDF file failed to open. You can refer to the API file for specific error
}
}.start()
}
}
<!-- Your activity_main.xml file -->
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<com.compdfkit.ui.reader.CPDFReaderView
android:id="@+id/readerview"
android:layout_width="match_parent"
android:layout_height="match_parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
- Run the application.
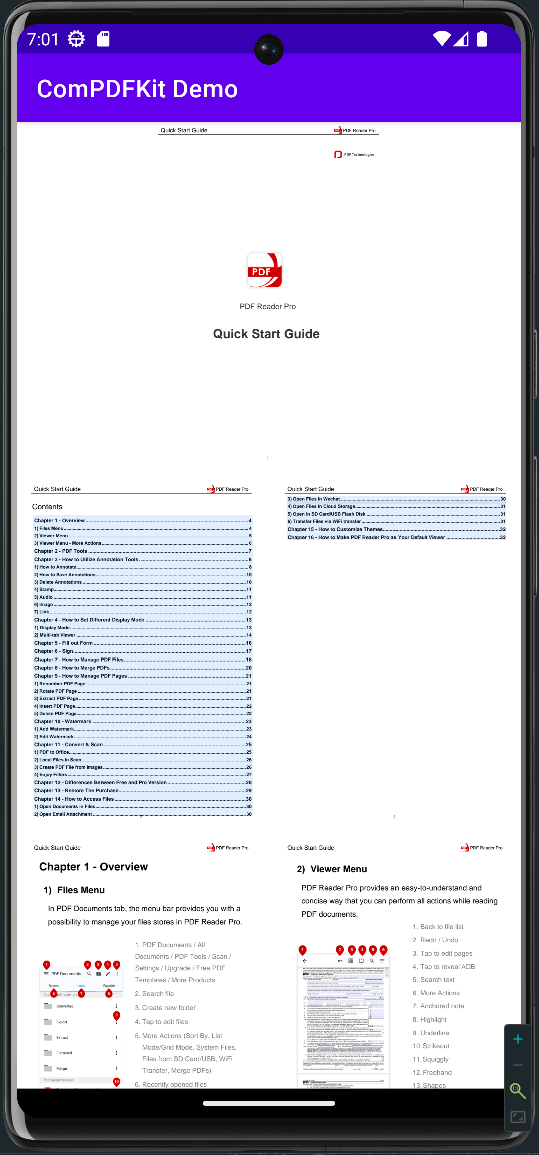
Now, with the help of ComPDFKit, you can get a simple application to display a PDF file.
Troubleshooting
Unable to Open the PDF Files
The license we provide to you is tied to your application ID, so ensure that the license acquired matches your application ID.
Other Problems
If you meet some other problems when integrating our ComPDFKit PDF SDK for Android, feel free to contact ComPDFKit team.