UI Customization
The folder of “ComPDFKit_Tools” includes the UI components to help conveniently integrate ComPDFKit PDF SDK. We have also built six standalone function programs, namely Viewer, Annotations, ContentEditor, Forms, DocsEditor, and Digital Signatures, using this UI component library. Additionally, we have developed a program called PDFViewer that integrates all the above-mentioned example features for reference.
In this section, we will introduce how to use it from the following parts:
- Overview of "ComPDFKit_Tools" Folder: Show the folder structure and the main features included in the corresponding component.
- UI Components: Introduce the UI components and how to use them easily and fast.
- Learn More: Show the special code structure and features for programming platforms.
Overview of "ComPDFKit_Tools" Folder
There are nine modules in "ComPDFKit_Tools": "Common", "Viewer", "Annotations", "ContentEditor", "Forms", "DocsEditor", "Digital Signatures", "Security", and "Watermark". Each of them includes the code and UI components like the following table to process PDFs.
Folder | Subfolder | Description |
---|---|---|
Common | - | Include the reusable UI components and interaction of Viewer, Annotations, ContentEditor, Forms, and DocsEditor. |
ContextMenu | Include the tool class of the PDF context menu. | |
Viewer | PDFBookmark | Include the UI components and interaction of editing bookmarks and jumping pages. |
PDFOutline | Include the UI components and interaction of jumping and displaying the PDF outline. | |
PDFSearch | Include the UI component and interaction for searching PDFs and generating the search list. | |
PDFDisplaySettings | Include the UI component and interaction of viewing PDFs. | |
PDFThumbnail | Include the UI component and interaction of PDF thumbnails. | |
Annotations | PDFAnnotationBar | Include the toolbar for choosing the annotation types, setting the properties of annotations, and undo/redo annotation property settings. |
PDFAnnatationList | Include the UI component and interaction of getting the annotation list. | |
PDFAnnotationProperties | Include the property panel and UI component of setting annotation properties. | |
ContentEditor | PDFEditBar | Include the toolbar to edit PDF text/images and undo/redo the processing of editing PDF text/images. |
PDFEditProperties | Include the UI component and interaction of PDF text and image editing. | |
Forms | PDFFormBar | Include the toolbar to set the properties of tables and undo/redo the processing of forms. |
PDFFormProperties | Include the property panel and interaction to set the properties of forms. | |
DocsEditor | PDFPageEdit | Include the UI component and interaction of the document editor. |
CPDFPageEditToolBar | Include the toolbar for creating, replacing, rotating, extracting, and deleting PDF pages. | |
PDFPageEditInsert | Include the UI component and interaction for creating blank pages and inserting other PDF pages. | |
Digital Signatures | CSignatureToolBar | Include the toolbar for creating form fields, filling out digital signatures, and verifying signatures. |
Security | CDocumentEncryptionDialog | Include the UI component and interaction of adding and removing the passwords to open documents or set file permissions. |
Watermark | CWatermarkEditDialog | Include the UI component and interaction of creating and editing the text and image watermark. |
UI Components
This section mainly introduces the connection between the UI components and API configuration of "ComPDFKit_Tools", which can not only help you quickly get started with the default UI but also help you view the associated API configuration. These UI components could be used and modified to create your customize UI.
The images below show the main UI components associated with the APIs of "ComPDFKit_Tools".
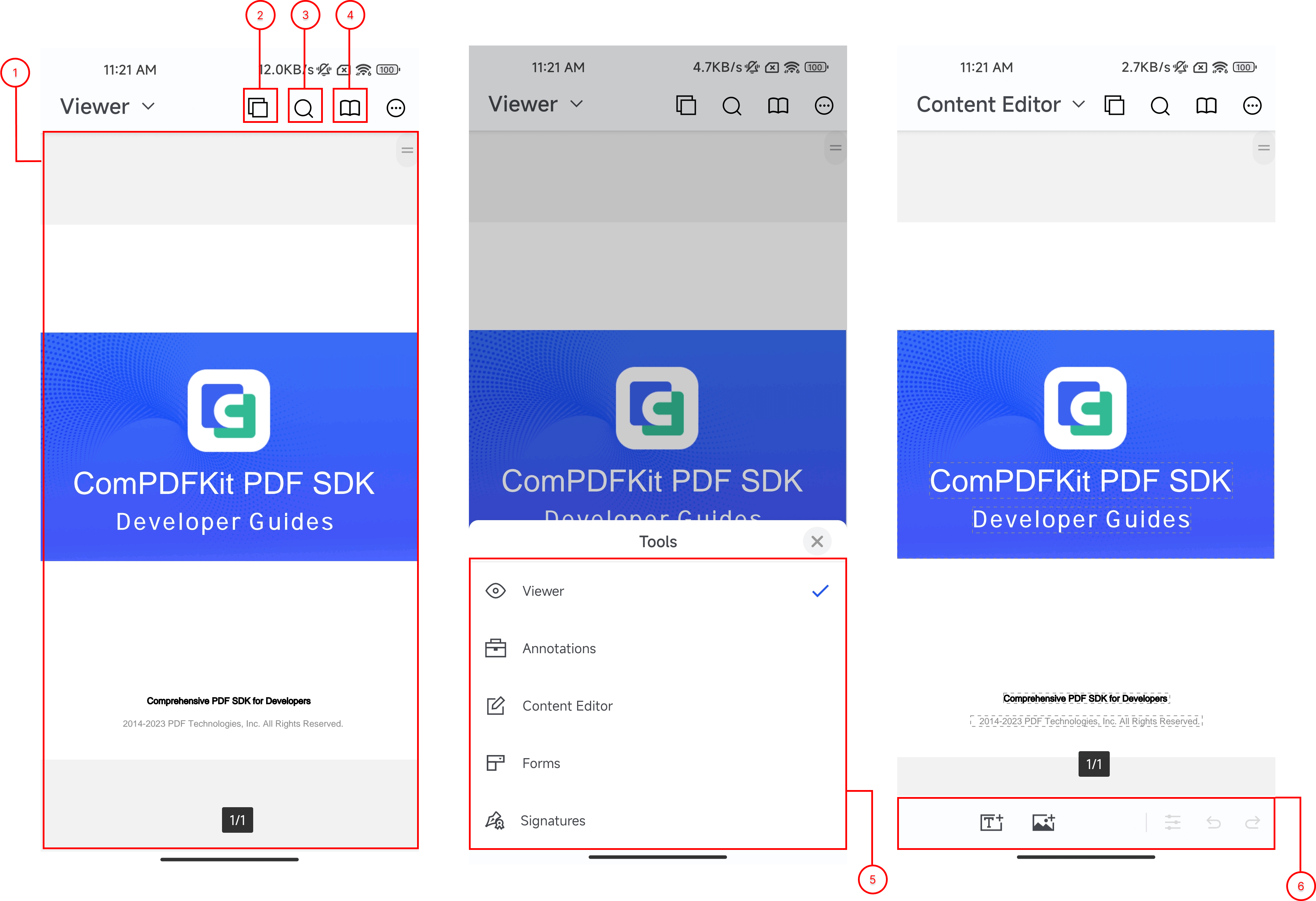
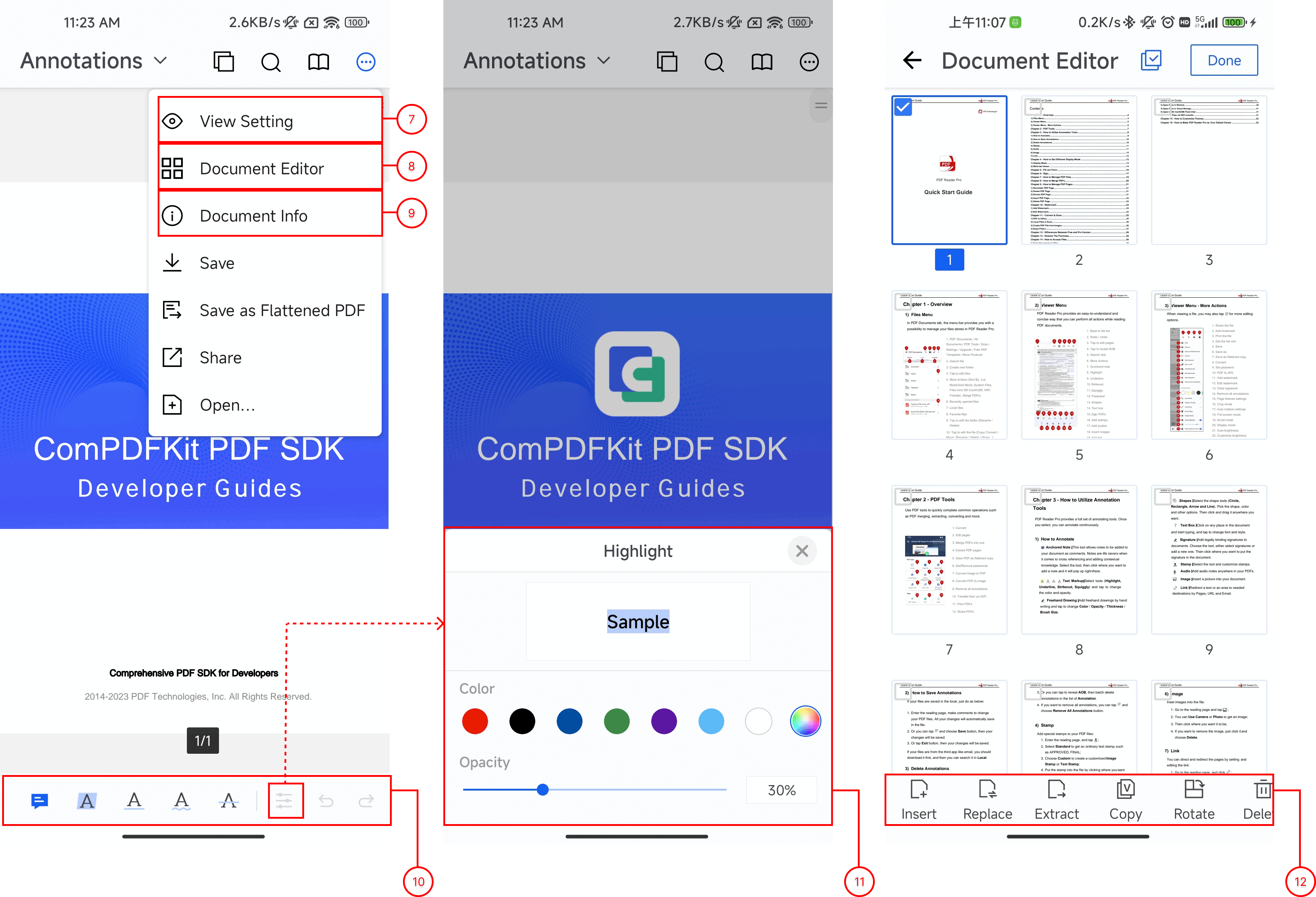
The following shows the details of the connection between UI components and APIs.
Number | Name | Functionality | Description |
---|---|---|---|
1 | PDFView | CPDFViewCtrl | CPDFViewCtrl is a ViewGroup that can be embedded into any layout. It includes a set of rich interactive PDF viewing features. |
2 | Thumbnails | CPDFThumbnailFragment | Enter the thumbnails of PDF pages. |
3 | Text search | CSearchToolbar | Enter the searching mode. |
4 | BOTA | CPDFBotaDialogFragment | Enter the list of outlines, bookmarks, and annotations. |
5 | Mode Switch | CModeSwitchDialogFragment | Switch the feature modules. |
6 | Edit toolbar | CEditToolbar | Set the properties of PDF text/images and undo/redo the processing of editing PDF content. |
7 | View setting | CPDFDisplaySettingDialogFragment | Enter the view setting of PDFs to set displaying mode, reading themes, splitting view, etc. |
8 | Page edit | CPDFPageEditDialogFragment | Enter the document pages editing of PDFs. |
9 | Document Info | CPDFDocumentInfoDialogFragment | Enter the information list of PDF files. |
10 | Annotation toolbar | CAnnotationToolBar | Set the properties of annotations and undo/redo the processing of annotations. |
11 | Properties | CStyleDialogFragment | Open the property panel. |
12 | PageEdit toolbar | CPageEditToolBar | Edit PDF pages. |
13 | digital signatures toolbar | CSignatureToolBar | The Digital Signature toolbar. |
14 | Customize signature preview | CDigitalSignStylePreviewDialog | Customize the digital signature appearance. |
15 | sign info | CertDigitalSignInfoDialog | Present the details of digital signatures. |
Learn More
The following part will introduce the frequently used UI components of the ”ComPDFKit_Tools“ module, to help you quickly familiarize yourself with the commonly used APIs and speed up the integration process.
Part 1. CPDFViewCtrl
Here are the examples of using CPDFViewCtrl
in MainActivity.java
.
- Add
CPDFViewCtrl
in layout.
<com.compdfkit.tools.common.views.pdfview.CPDFViewCtrl
android:id="@+id/pdf_view"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:tools_enable_page_indicator="true"
app:tools_enable_slider_bar="true"
app:tools_page_indicator_margin_bottom="20dp"
app:tools_slider_bar_icon="@drawable/tools_ic_pdf_slider_bar"
app:tools_slider_bar_position="right" />
- Get
CPDFViewCtrl
public class CMainActivity extends AppCompatActivity {
@Override
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.pdf_sample_activity);
CPDFViewCtrl pdfView = findViewById(R.id.pdf_view);
}
}
class CMainActivity : AppCompatActivity() {
override fun onCreate(@Nullable savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.pdf_sample_activity)
val pdfView: CPDFViewCtrl = findViewById(R.id.pdf_view)
}
}
- Load the files by links or file path
//Use Uri
pdfView.openPDF(Uri pdfUri);
//Use file Path
pdfView.openPDF(String filePath);
Note: If you want to open an encrypted PDF, a popup window will pop out for you to type in the passwords. Check CVerifyPasswordDialogFragment
for details.
Part 2. PDF Properties
It's a common feature to modify properties of objects such as Annotations, Forms, etc. In the "ComPDFKit_Tools" module, we've encapsulated these features, please refer to the following examples.
- Set Default Properties
This section will demonstrate how to set default property values. Take highlight annotations as an example:
//Set default properties without property panel
new CStyleManager.Builder()
.setMarkup(CStyleType.ANNOT_HIGHLIGHT, color, colorAlpha)
.init(CPDFViewCtrl, true);
//Set default properties without property panel
CStyleManager.Builder()
.setMarkup(CStyleType.ANNOT_HIGHLIGHT, color, colorAlpha)
.init(pdfViewCtrl, true)
If you need to use the property panel to change attribute values globally, such as selecting highlight annotation in the CAnnotationToolBar
UI component and clicking the Setting button on the right to modify the default value of highlight annotation, you can refer to the following sample code.
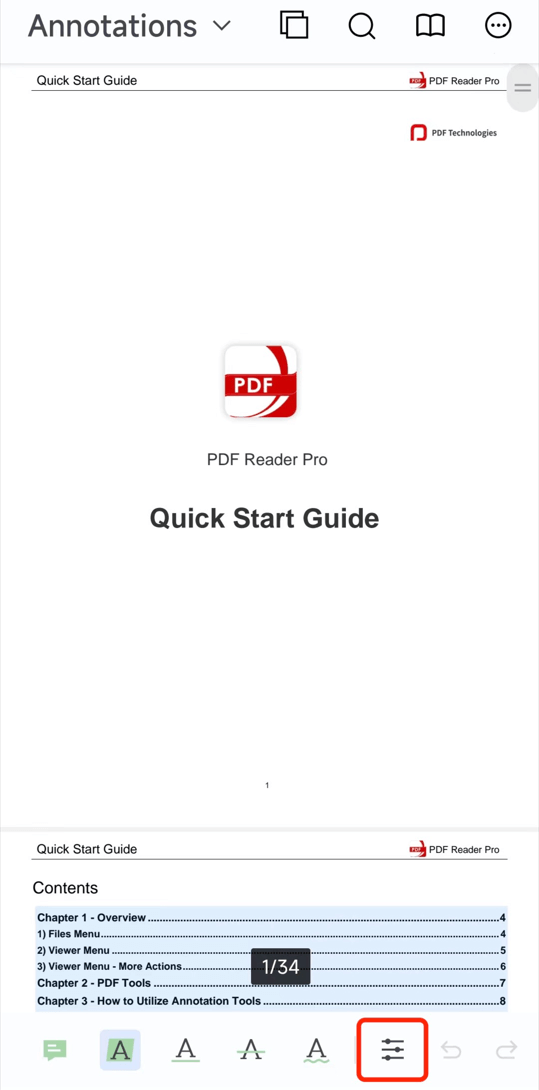
//Create manage class: CStyleManager
CStyleManager styleManager = new CStyleManager(pdfView);
//Get current highlight properties
CAnnotStyle style = styleManager.getStyle(CStyleType.ANNOT_HIGHLIGHT);
//Create popup windows: Style
CStyleDialogFragment dialogFragment = CStyleDialogFragment.newInstance(style);
//Set up the data listener of the property panel, global attribute values will be modified in this method when adjusting colors and transparency.
styleManager.setAnnotStyleFragmentListener(dialogFragment);
dialogFragment.show(fragmentManager, "annotStyleDialogFragment");
//Create manage class: CStyleManager
val styleManager = CStyleManager(pdfView)
//Get current highlight properties
val style: CAnnotStyle = styleManager.getStyle(CStyleType.ANNOT_HIGHLIGHT)
//Create popup windows: Style
val dialogFragment = CStyleDialogFragment.newInstance(style)
//Set up the data listener of the property panel, global attribute values will be modified in this method when adjusting colors and transparency.
styleManager.setAnnotStyleFragmentListener(dialogFragment)
dialogFragment.show(fragmentManager, "annotStyleDialogFragment")
Note: For retrieving or setting attribute values from ComPDFKit PDF SDK, you could refer to the updateStyle()
and getStyle()
methods in CGlobalStyleProvider.java
class.
- Modify the properties of the selected annotations or forms
When you select an annotation, you can get the selected annotation in CPDFContextMenuHelper.java
class. You can get and modify the properties from the selected annotation. The following will take the selected highlight annotation as an example to show you how to change the properties of selected annotations.
Please make sure you have the permission to edit annotations.
// You can set the mode to ViewMode.ANNOT or ViewMode.ALL, in both modes you can select annotations.
pdfView.setViewMode(CPDFReaderView.ViewMode.ANNOT);
Please ensure that setContextMenuShowListener
is set, you can use the default context menu provided by "ComPDFKit_Tools".
pdfView.getCPdfReaderView().setContextMenuShowListener(
new CPDFContextMenuHelper.Builder().defaultHelper()
.create(pdfView));
pdfView.getCPdfReaderView().setContextMenuShowListener(
CPDFContextMenuHelper.Builder().defaultHelper()
.create(pdfView))
After selecting the highlight annotation, you can get the highlight annotation object CPDFBaseAnnotImpl
in the method getMarkupContentView()
of CPDFContextMenuHelper
class.
public class CPDFContextMenuHelper extends CPDFContextMenuShowHelper {
//...
@Override
public View getMarkupContentView(CPDFPageView cpdfPageView, CPDFBaseAnnotImpl cpdfBaseAnnot, LayoutInflater layoutInflater) {
//getMarkupContentView includes Highlight, Underline, Strikeout, and Squiggly.
ContextMenuView menuView = new ContextMenuView(readerView.getContext());
menuView.addItem(R.string.tools_context_menu_properties, v -> {
//Create the managing class of properties.
CStyleManager styleManager = new CStyleManager(cpdfBaseAnnot, cpdfPageView);
//Get all the properties of highlight annotations.
CAnnotStyle annotStyle = styleManager.getStyle(CStyleType.ANNOT_HIGHLIGHT);
//Create the property panel
CStyleDialogFragment styleDialogFragment = CStyleDialogFragment.newInstance(annotStyle);
//Add Listener for Property Panel in Management Class.
styleManager.setAnnotStyleFragmentListener(styleDialogFragment);
//When the property panel pops up, offset CPDFReaderView to keep the selected annotation appearing above the pop-up window.
styleManager.setDialogHeightCallback(styleDialogFragment, readerView);
styleDialogFragment.show(((FragmentActivity) readerView.getContext()).getSupportFragmentManager(), "noteEditDialog");
dismissContextMenu();
});
return menuView;
}
}
```tar xzvf archive.tar.gz
```kotlin
class CPDFContextMenuHelper(readerView: CPDFReaderView) : CPDFContextMenuShowHelper(readerView) {
//...
override fun getMarkupContentView(cpdfPageView: CPDFPageView, cpdfBaseAnnot: CPDFBaseAnnotImpl<*>?, layoutInflater: LayoutInflater): View {
//getMarkupContentView includes Highlight, Underline, Strikeout, and Squiggly.
val menuView = ContextMenuView(readerView.context)
menuView.addItem(R.string.tools_context_menu_properties) { v: View? ->
//Create the managing class of properties.
val styleManager = CStyleManager(cpdfBaseAnnot, cpdfPageView)
//Get all the properties of highlight annotations.
val annotStyle = styleManager.getStyle(CStyleType.ANNOT_HIGHLIGHT)
//Create the property panel
val styleDialogFragment = CStyleDialogFragment.newInstance(annotStyle)
//Add Listener for Property Panel in Management Class.
styleManager.setAnnotStyleFragmentListener(styleDialogFragment)
//When the property panel pops up, offset CPDFReaderView to keep the selected annotation appearing above the pop-up window.
styleManager.setDialogHeightCallback(styleDialogFragment, readerView)
styleDialogFragment.show((readerView.context as FragmentActivity).supportFragmentManager, "noteEditDialog")
dismissContextMenu()
}
return menuView
}
}
Note:
- You can check out the
CSelectedAnnotStyleProvider
class to learn how to set and get the properties of selected annotations and forms with ComPDFKit PDF SDK. - No matter what kind of properties you need to modify, you can do that through the
CStyleDialogFragment
property panel and theCStyleManager
class.
Part 3. CPDFContextMenuHelper
The "ComPDFKit_Tools" module provides you with the CPDFContextMenuHelper
class. With this class, you can easily customize the context menu for annotations. Of course, we also provide you with default context menu classes. The following shows you how to use the default context menu classes.
- use the default context menu classes
CPDFContextMenuHelper
.
pdfView.getCPdfReaderView().setContextMenuShowListener(
new CPDFContextMenuHelper.Builder().defaultHelper()
.create(pdfView));
pdfView.getCPdfReaderView().setContextMenuShowListener(
CPDFContextMenuHelper.Builder().defaultHelper()
.create(pdfView))
- Customize the context menu. The following code will show you how to customize the context menu with the example of highlight annotations.
Implement the ContextMenuMarkupProvider
class which is provided by the "ComPDFKit_Tools" module.
public class CMarkupContextMenuView implements ContextMenuMarkupProvider {
@Override
public View createMarkupContentView(CPDFContextMenuHelper helper, CPDFPageView pageView, CPDFMarkupAnnotImpl markupAnnotImpl) {
ContextMenuView menuView = new ContextMenuView(helper.getReaderView().getContext());
//Add the button of properties.
menuView.addItem(R.string.tools_context_menu_properties, v -> {
CStyleManager styleManager = new CStyleManager(markupAnnotImpl, pageView);
CAnnotStyle annotStyle = styleManager.getStyle(CStyleType.ANNOT_HIGHLIGHT);
CStyleDialogFragment styleDialogFragment = CStyleDialogFragment.newInstance(annotStyle);
styleManager.setAnnotStyleFragmentListener(styleDialogFragment);
styleManager.setDialogHeightCallback(styleDialogFragment, helper.getReaderView());
if (helper.getReaderView().getContext() instanceof FragmentActivity) {
styleDialogFragment.show(((FragmentActivity) helper.getReaderView().getContext()).getSupportFragmentManager(), "noteEditDialog");
}
helper.dismissContextMenu();
});
//Add the button for note annotations.
menuView.addItem(R.string.tools_annot_note, v -> {
CPDFAnnotationManager annotationManager = new CPDFAnnotationManager();
annotationManager.editNote(helper.getReaderView(), pageView, markupAnnotImpl.onGetAnnotation());
helper.dismissContextMenu();
});
//Add the button to delete annotations.
menuView.addItem(R.string.tools_delete, v -> {
pageView.deleteAnnotation(markupAnnotImpl);
helper.dismissContextMenu();
});
return menuView;
}
}
class CMarkupContextMenuView : ContextMenuMarkupProvider {
override fun createMarkupContentView(helper: CPDFContextMenuHelper, pageView: CPDFPageView, markupAnnotImpl: CPDFMarkupAnnotImpl): View {
val menuView = ContextMenuView(helper.readerView.context)
//Add the button of properties.
menuView.addItem(R.string.tools_context_menu_properties) {
val styleManager = CStyleManager(markupAnnotImpl, pageView)
val annotStyle = styleManager.getStyle(CStyleType.ANNOT_HIGHLIGHT)
val styleDialogFragment = CStyleDialogFragment.newInstance(annotStyle)
styleManager.setAnnotStyleFragmentListener(styleDialogFragment)
styleManager.setDialogHeightCallback(styleDialogFragment, helper.readerView)
if (helper.readerView.context is FragmentActivity) {
styleDialogFragment.show((helper.readerView.context as FragmentActivity).supportFragmentManager, "noteEditDialog")
}
helper.dismissContextMenu()
}
//Add the button for note annotations.
menuView.addItem(R.string.tools_annot_note) {
val annotationManager = CPDFAnnotationManager()
annotationManager.editNote(helper.readerView, pageView, markupAnnotImpl.onGetAnnotation())
helper.dismissContextMenu()
}
//Add the button to delete annotations.
menuView.addItem(R.string.tools_delete) {
pageView.deleteAnnotation(markupAnnotImpl)
helper.dismissContextMenu()
}
return menuView
}
}
Apply the custom highlight annotation
context menu. You can also refer to the CPDFContextMenuHelper.Builder()
class to customize more other context menus.
pdfView.getCPdfReaderView().setContextMenuShowListener(
new CPDFContextMenuHelper.Builder()
.setMarkupContentMenu(new CMarkupContextMenuView())
.create(pdfView));
pdfView.getCPdfReaderView().setContextMenuShowListener(
CPDFContextMenuHelper.Builder()
.setMarkupContentMenu(CMarkupContextMenuView())
.create(pdfView))